Optimizing Firmware Performance for Resource-Constrained Devices

Optimizing Firmware Performance for Resource-Constrained Devices
When developing firmware for resource-constrained devices like microcontrollers, performance optimization becomes critical. Unlike desktop or server applications, embedded systems often operate with limited CPU power, memory, and energy resources. This article explores practical techniques for squeezing maximum performance out of minimal hardware.
Understanding the Constraints
Before optimizing, it’s essential to understand your specific constraints:
- CPU speed and architecture: Different microcontroller families have vastly different performance characteristics
- Memory constraints: Both program memory (flash) and RAM limitations
- Power requirements: Battery-powered devices need to balance performance with energy efficiency
- Real-time requirements: Many embedded applications have strict timing constraints
Optimization strategies should address your most pressing constraints while making appropriate trade-offs.
Memory Optimization Techniques
Program Memory (Flash) Optimization
- Use appropriate data types: Choose the smallest data type that meets your needs
- Eliminate dead code: Use link-time optimization to remove unused functions
- Minimize string usage: String literals consume flash memory
- Consider compression techniques: For large data tables or resources
RAM Optimization
- Stack analysis: Determine maximum stack usage and minimize local variables
- Static vs. dynamic allocation: Prefer static allocation when possible
- Buffer sharing: Reuse buffers for operations that don’t overlap in time
- Packed structures: Use bit fields and careful structure organization
Execution Speed Optimization
- Algorithmic efficiency: Choose algorithms appropriate for your constraints
- Compiler optimization flags: Understanding and properly using optimization levels
- Inline critical functions: When appropriate for your architecture
- Loop unrolling: For small, critical loops
- Lookup tables vs. computation: Trading memory for speed
- Assembly optimization: For the most critical sections only
Power Optimization
- Sleep modes: Effective use of different power states
- Peripheral management: Disable unused peripherals
- Clock management: Run at the lowest clock speed that meets requirements
- Burst processing: Process data in bursts, then sleep
- DMA utilization: Offload data transfer from the CPU
Measuring and Profiling
You can’t optimize what you don’t measure. Effective optimization requires:
- Baseline measurements: Establish performance before optimization
- Targeted profiling: Identify the most resource-intensive parts of your code
- Verification: Ensure optimizations don’t break functionality
- Iterative approach: Make one change at a time and measure impact
Case Study: Sensor Data Processing
In a recent project, we needed to process sensor data on an STM32 microcontroller with tight power constraints. Initial implementation consumed too much power and missed data points during peak processing.
Our optimization approach:
- Profiled the code to identify processing bottlenecks
- Replaced floating-point calculations with fixed-point arithmetic
- Implemented a circular buffer to batch sensor readings
- Used DMA for sensor data acquisition
- Optimized the main processing loop in assembly
The result was a 65% reduction in processing time and 40% lower power consumption, meeting all project requirements.
Conclusion
Optimizing firmware for resource-constrained devices requires a systematic approach and deep understanding of your specific constraints. By methodically analyzing your system, measuring performance, and applying targeted optimizations, you can achieve remarkable efficiency even on the most limited hardware platforms.
Remember that premature optimization is indeed the root of many problems—optimize where it matters, based on data, not assumptions.
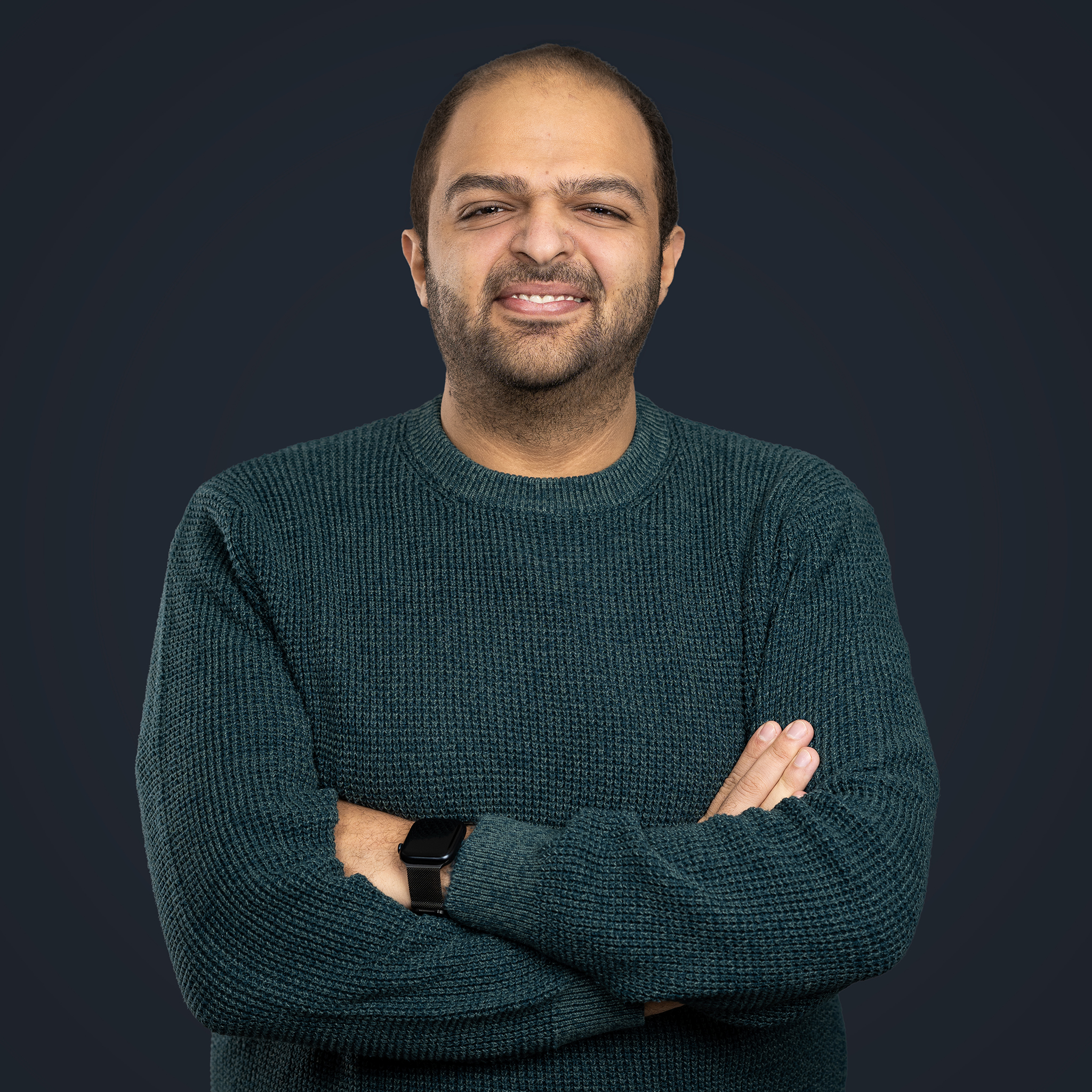
Ahmed Tourk
Engineering manager and embedded software engineer with over 10 years of experience building innovative solutions and leading high-performance teams.